Introduction
To be a hirable developer, you need to know a framework. But how do you know when you are ready to learn one?
In this article, tech industry Masters Matt Lawrence and Mike Karan will guide you in effectively learning the essential JavaScript fundamentals required before learning a framework. Through these JavaScript trials, you will build a strong foundation that will set you up for success in learning a framework, ultimately providing you with a competitive advantage in joining the employed side of the Workforce!
Topics covered in this article include:
- Importance of learning JavaScript before a framework
- Essential JavaScript concepts to know before learning a framework
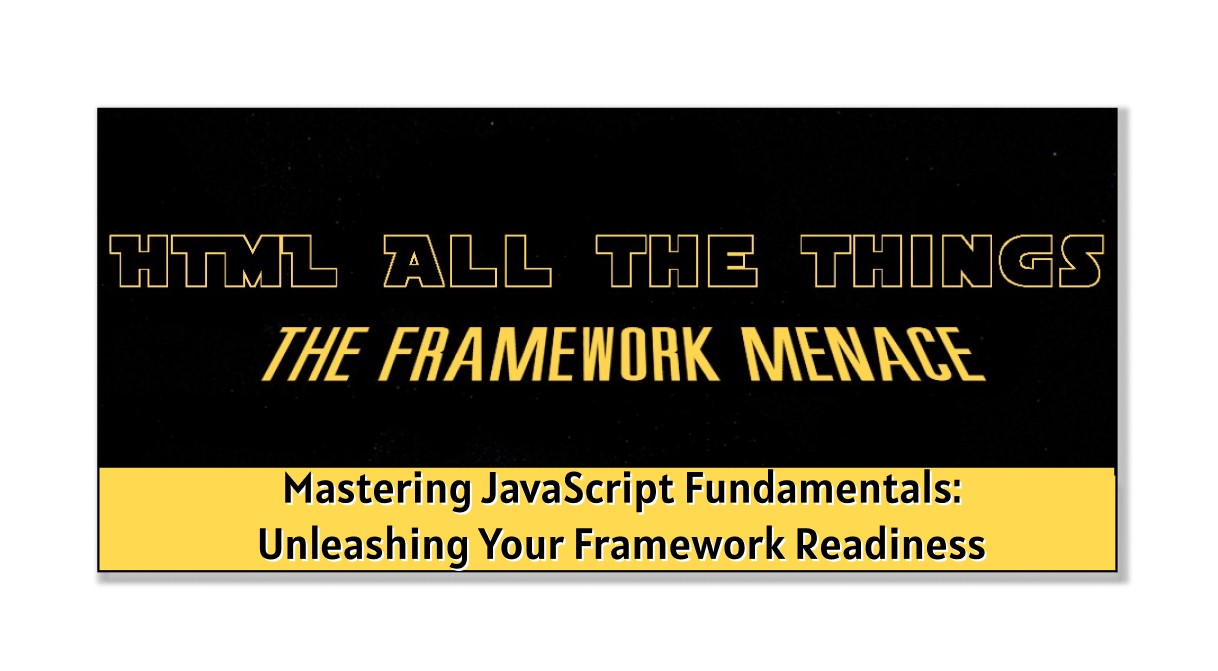
Why is it important to learn JavaScript before learning a framework?
A framework, such as React, is built "on top of" JavaScript, which means that React is a library that extends and utilizes JavaScript's core features.
We use React as a tool to help build web pages with interactive elements more easily by creating reusable parts for websites and managing how information is displayed. Using a framework also makes it easier to create and maintain large projects.
Learning JavaScript before diving into a framework is important for several reasons. First, you must grasp the essential programming concepts and fundamentals, as these as also relevant in frameworks. In addition to knowing and recognizing JavaScript syntax, you must be able to effectively solve problems with the JavaScript language (a programmer is, first and foremost, a problem solver)!
When you are well-versed in JavaScript, it will be much easier for you to pick up a framework such as React. Not only will you be able to differentiate vanilla JavaScript code from React code, but you will also be able to differentiate errors, enabling you to resolve problems more quickly.
Essential Benefits of Learning JavaScript Before Frameworks include:
- Debugging: Easier identification and resolution of errors in code when familiar with JavaScript fundamentals.
- Code differentiation: Recognizing the differences between vanilla JavaScript and framework-specific code, enabling more efficient problem-solving.
- Customization: Ability to modify and extend framework functionality when necessary due to a deep understanding of the underlying JavaScript.
- Performance optimization: Knowledge of JavaScript helps in optimizing code for better performance within a framework.
- Transferable skills: Proficiency in JavaScript fundamentals allows for easier learning and adaptation to different frameworks and libraries.
So, as a JavaScript developer, what is a good use case example for your first framework-based project? A to-do list app is a good use case for utilizing a framework like React over vanilla JavaScript. With React, you can more easily build and maintain a to-do list app that allows users to add, edit, and delete list items. Trying to code a project like this in vanilla JavaScript would be much more complicated.
What do you need to know before learning a framework?
Since developer jobs mostly require framework knowledge, it will be tempting for you to rush into learning it if your intent is employment.
Don’t be tempted by the power of the framework! Taking the quick and easy path of rushing through your JavaScript training will lead you down the path of the unemployed side of the Workforce! Only a fully trained JavaScript developer can wield the true power of a framework!
So, how will you know when you are ready to face the JavaScript trials and thereafter learn a framework to become a front-end developer? To become a proficient front-end web developer skilled in using frameworks, a JavaScript developer must first embark on a series of challenges that test their mastery of JavaScript fundamentals.
These trials are designed to evaluate a developer's comprehension of programming concepts. Only when you truly comprehend and can effectively utilize these programming concepts will you be prepared to harness the power of a framework, ultimately becoming a skilled front-end web developer who is well-equipped for the employed side of the Workforce!
Mike and Matt established the following foundation for these JavaScript trials.
The fundamentals JavaScript concepts you need to know:
- Variables
- const, let, var - Conditional Statements
- if/else
- switch
- ternary
- optional chaining
- conditionals for display and not display are major parts of frameworks - Scoping
- Looping
- while
- for - Arrays
- for each
- map
- reduce
- filter
- sort - Async/await, promises, fetch
- Getting, waiting for, and propagating data is a big part of why we use frameworks - Template Literals
- Frameworks like React can benefit a lot from combining strings, functions, and variables in the same line - especially for templating - Deconstructing
- Spread operator
We will take a closer look at each of the fundamental JavaScript concepts in the following article sections.
Variables
JavaScript has three variable declarations: const, let, and var.
- const is used for constant values that cannot be reassigned after the declaration
- let is used for variables with block scope, which can be reassigned
- var is used for function-scoped variables that can also be reassigned
However, beware of the var variable! Using var has some shortcomings: it has function scope rather than block scope, which can lead to unintended variable hoisting and confusion. This is why it is advisable to use let and const, which have block scope and provide better control over variable scoping and mutability.
The following code block initializes a constant variable fullname with the value "Luke Skywalker" and a let variable weapon with the value "DL-44 blaster". Then, it reassigns the value of weapon to "Lightsaber".
As fullname is a const variable, it can not be reassigned!
Conditional statements
Conditional statements are essential constructs in programming that allow developers to control the flow of their code based on specific conditions.
This section will explore different types of conditional statements in JavaScript, such as if/else, switch, ternary, optional chaining, and conditionals for display and not display, commonly used in frameworks.
- if/else: A basic conditional structure that executes one block of code if a condition is true, and another block if it's false.
- switch: A conditional structure that checks a given value against multiple cases and executes the associated block of code.
- ternary: A shorthand conditional expression that returns one of two values, depending on the result of a condition.
- optional chaining: A syntax feature that allows safe property access when a value might be undefined or null.
- conditionals for display and not display: Techniques used in frameworks to conditionally render or hide elements based on specific conditions.
if/else
The following code block defines a function called chooseSide that takes an argument 'side.' If the value of 'side' is "dark," it returns a string indicating that Anakin Skywalker chooses the Dark Side of the Force and becomes Darth Vader. If the value of 'side' is anything other than "dark," it returns a string indicating that Anakin chooses the Light Side of the Force and remains Anakin Skywalker.
switch
This code block defines a function called getCharacterQuote that takes a character name as an argument and returns a famous quote associated with that character from the Star Wars universe. If the character name does not match any of the cases, it returns the default quote, "May the Force be with you."
ternary
The following code initializes a boolean variable didHanSoloShootFirst as true. It then defines two string variables, paragraphHanShotFirst and paragraphHanShotSecond, which contain paragraphs describing the two different versions of the cantina scene in Star Wars Episode IV: A New Hope.
The ternary conditional expression at the end checks the value of didHanSoloShootFirst. If true, it returns the paragraphHanShotFirst string. Otherwise, it returns paragraphHanShotSecond.
Syntax: ifTrueOrFalse ? resultIfTrue : resultIfFalse;
optional chaining
Optional chaining is a feature in JavaScript that allows you to safely access the properties of an object, even if the object or its properties might be undefined or null. It helps prevent errors that could occur when trying to access non-existent properties.
The following code block defines an object called starWarsCharacters with three properties, each representing a character from the Star Wars universe. Each character has their occupation and weapon as nested properties.
Then, it attempts to find the weapon of a character named Baylan Skoll. If the character is not found, the baylanSkollsWeapon variable will be assigned the value "Unknown weapon."
conditionals for display and not display
Conditionals for display and not display are techniques used in web development to show or hide elements on a web page based on specific conditions. This helps create dynamic and interactive user interfaces by controlling which content is visible to users under different circumstances.
The following React code defines a React functional component called StarWarsCharacter that displays information about a character named Din Djarin. It uses useState hook to manage a boolean state, displayInfo, for toggling the visibility of the character's information.
When the button is clicked, it toggles the displayInfo state, and the character's information is conditionally rendered based on the value of displayInfo.
Scoping
Scoping in JavaScript refers to the accessibility and lifetime of variables within different parts of your code.
There are two main types of scoping:
- Global scope: Variables accessible throughout the entire codebase.
- Local scope: Variables restricted to a specific function or block of code.
Global scope means a variable is accessible throughout the entire codebase, while local scope restricts a variable's accessibility to a specific function or block of code. Understanding scoping is essential for managing variables effectively and avoiding potential conflicts or unintended behavior in your programs.
In the following code block, the variable "planet" is assigned the value "Coruscant" within the function scope, making it inaccessible outside of the function scope.
Looping
Looping in JavaScript allows you to execute a block of code repeatedly until a specified condition is met. There are several types of loops in JavaScript, including for, while, and do-while loops.
- for: The most commonly used loop, consisting of an initialization, a condition, and an update, which executes a block of code as long as the condition is true.
- while: Executes a block of code as long as the specified condition is true. The condition is checked before each iteration.
- do-while: Similar to a while loop, but the condition is checked after each iteration, ensuring the block of code is executed at least once.
Understanding loops in JavaScript is crucial for efficiently executing repetitive tasks and processing extensive datasets or collections.
The following code block demonstrates each loop type to iterate through an array of fighter spacecraft.
Arrays
In JavaScript, arrays are used to store collections of items, such as characters, numbers, or objects. They provide an organized way to manage and access data using indexes, representing each item's position in the array.
This code block example creates an array of bounty hunters and demonstrates how to access and manipulate the array elements.
Async/await, promises, fetch
Async/await, promises, and fetch are essential concepts in JavaScript for handling asynchronous operations, such as fetching data from an API.
In this section, we will illustrate the utilization of async/await, promises, and fetch for retrieving data from the Star Wars API.
This code block defines an async function called fetchStarWarsPlanets. Inside the function, we use fetch to request data from the Star Wars API for planets.
We then use await to wait for the response and convert it to JSON format. After that, we extract the planet names from the JSON data and log them to the console. If there's an error during the process, we catch it and log the error message.
Finally, we call the fetchStarWarsPlanets function to execute the code.
Comparing String Concatenation and Template Literals
The following code block demonstrates two ways of combining strings to create a new string. Both methods produce the same result but with different syntax.
The variable resultOne uses string concatenation with the + operator. It combines the values of verbOne, nounOne, and nounTwo with additional strings to form a complete sentence.
The variable resultTwo uses template literals with backticks (`) and ${}. It embeds the variables directly in the string, making the code more readable and easier to maintain.
Both console.log() statements print the same sentence, "May the Force be with you"
Deconstructing
Deconstructing, also known as destructuring, is a concise way to extract values from objects and arrays in JavaScript. It allows you to assign values to variables directly from an object or an array, making your code more readable and efficient.
Understanding deconstructing in JavaScript enables you to write cleaner and more efficient code when working with objects and arrays.
The following code example demonstrates object destructuring to extract information about a character and array destructuring to access vehicle names.
Spread operator
The spread operator in JavaScript, represented by three dots (...) is used to expand the elements of an array, object, or string. It can be helpful for tasks like merging arrays, cloning objects, and passing function arguments.
The spread operator allows you to work with arrays and objects more efficiently and flexibly.
This code block demonstrates the use of the spread operator to combine the forces of the Galactic Empire and the First Order.
Resources
Now that Mike and Matt have established the groundwork for you to build a strong foundation in the JavaScript fundamentals, setting you up for success in learning a framework, it's time for you to get plenty of hands-on practice through the JavaScript trials!
Recommended resources for JavaScript trials:
- freeCodeCamp: An online learning platform that offers free coding lessons and projects to help you master JavaScript fundamentals and other web development technologies.
- edabit: A coding challenge platform where you can practice JavaScript through small, manageable exercises designed to help you improve your problem-solving skills.
- iCodeThis: A web-based platform that provides a variety of coding exercises and challenges, including JavaScript, to help you sharpen your skills and enhance your understanding of programming concepts.
- Scrimba: An interactive learning platform that offers hands-on coding tutorials and courses, including JavaScript and web development, allowing you to learn by doing and receive instant feedback.
Quincy Larson founded freeCodeCamp, a nonprofit online learning platform that offers coding lessons and projects in web development, including JavaScript, HTML, CSS, the React framework, and more. Not only will you get plenty of hands-on coding practice, but you will also earn certifications! I earned the Responsive Web Design Certification and can highly recommend learning with them! You can learn more by reading my article Learn to code and earn a certification for free.
The edabit website is an excellent place to practice JavaScript. You can choose a coding language ( including JavaScript ), set a difficulty level, and gain experience points to level up, just like a fun game! Your progress is also tracked and displayed on a GitHub-style square commit grid. They also provide beginner-friendly algorithm challenges!
The iCodeThis website is a new up-and-coming platform created by tech celebrity Florin Pop! The iCodeThis platform offers daily coding challenges, a place to code original projects to store and share your work, and leaderboards, encouraging you to do your best to make the ranks and cheer on your fellow iCoders in friendly competitions! To learn more, you can read my review article iCodeThis: Improve Your Coding Skills With Free Daily Challenges.
Scrimba is much more than just an online coding school. They boast an incredible staff and teachers, supportive students, and a fantastic community, making learning an immersive experience. Scrimba not only teaches you how to code, but they also help you to confidently develop your skillset and effectively prepare for the job market by helping you with interview preparation, your resume, LinkedIn, and more! I've been personally helped on several YouTube Livestream Scrimba events and have written over 50 articles covering my positive experiences with them!
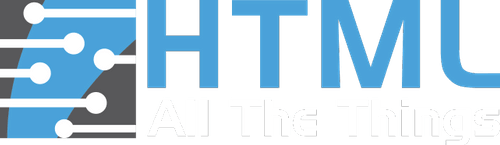
Be sure to listen to the episode!
Episode 237 JavaScript Fundamentals You Need to Know Before Learning React
Be sure to check out HTML All The Things on socials!
Scrimba Discount!
- Learn to code using Scrimba with their interactive follow-along code editor
- Join their exclusive discord communities and network to find your first job!
- Use this URL to get 10% off on all their paid plans: https://tinyurl.com/ScrimbaHATT
My other related articles
- JavaScript: How to Use the Star Wars API for Beginners
- React: How to Use the Star Wars API for Beginners
- Han Solo - Creating a True/False Toggle in React with useState Hook for Beginners
- Learn Local Storage in React: Star Wars Light and Dark Theme Switcher Application
The Star Wars content in this article is utilized for educational and informative purposes under fair use. Enjoy the learning journey!
Conclusion
Mastering the JavaScript fundamentals will prepare you for the next step of learning and harnessing the true power of frameworks.
Only a programmer that truly grasps the essential JavaScript concepts and fundamentals will be strong enough in coding to wield the power of a framework! Rember, a JavaScript programmer uses their skills to solve problems, not to create errors!
As you embark on this epic journey, remember that patience, dedication, and a strong foundation in both JavaScript and frameworks will lead you to become a fully trained front-end developer ready to face the challenges of the tech industry.
May the employed side of the Workforce be with you!
Let's connect! I'm active on LinkedIn and Twitter.
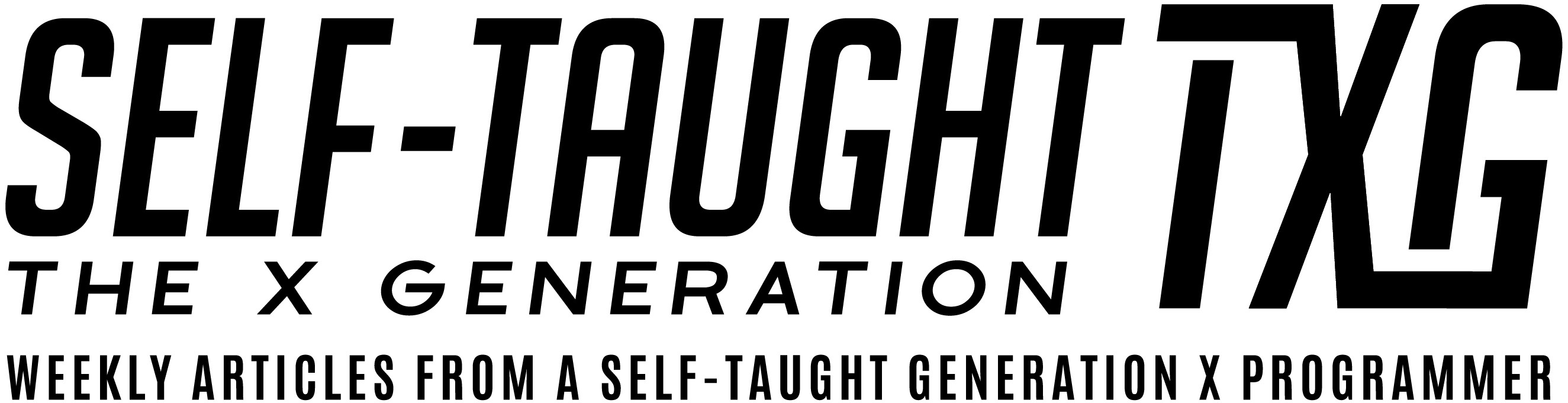
You can read all of my articles on selftaughttxg.com